【python】OpenCV画像処理方法(画像表示、データ格納、サイズ変更、回転)
Python-OpenCV
pip install python-opencv
python版のopencvのライブラリをインストールしておく。
画像
画像を表示する。
import cv2 # 画像選択 img = cv2.imread('sample.jpg') # 画像表示 cv2.imshow('OpenCV', img) # 表示 cv2.waitKey(0) cv2.destroyAllWindows() # 閉じる
画像データの確認
今回は、下記の画像(横×縦:1280×887)を使用したいと思います。
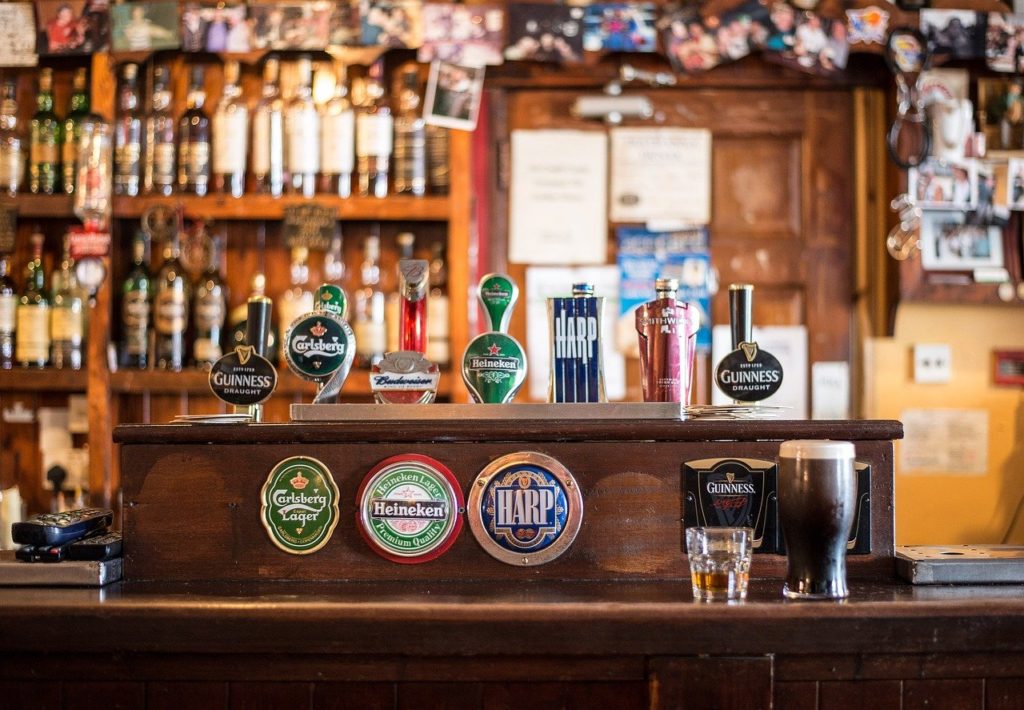
注意点として、OpenCVで画像を扱う場合、画像のサイズ表示は、縦×横の順番で表記します。
画像の1画素(pixel)のBGR情報確認は、下記のような配列の値を取得する形で、できます。
print(img[0, 0]) # 画像の左上 print(img[886, 0]) # 画像の左下 print(img[0, 1279]) # 画像の右上 print(img[886, 1279]) # 画像の右下 # --出力結果-- # [12 38 68] # [ 2 2 16] # [154 153 162] # [ 6 9 30]
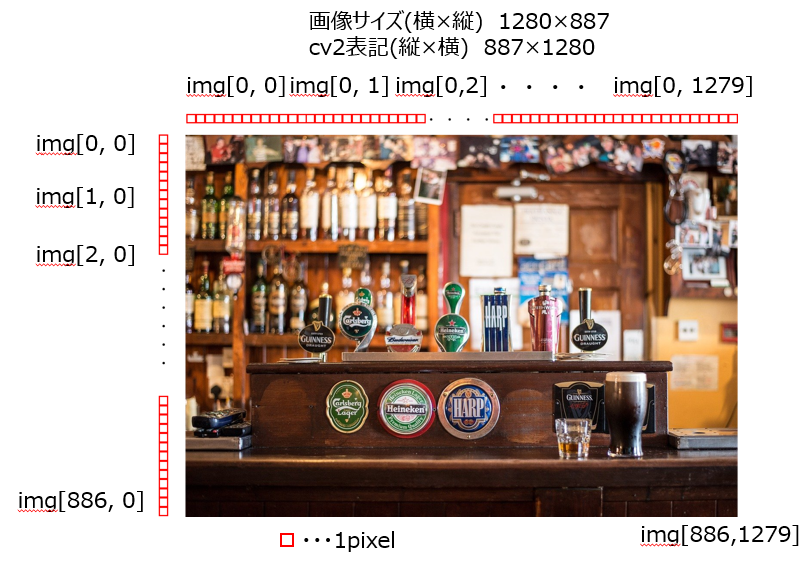
画像サイズ変更
まずは、画像のwidthとheightの取得方法です。
# 画像のサイズを変更 height, width, channels = img.shape print(width, height) #--出力結果-- # 1280 887
画像の縮小と拡大する方法。
# 画像のサイズ変更(pixel数値指定) # サイズの形状は、縦×横だが、サイズ変更時は、横×縦で指定する形となる。 img_resized1 = cv2.resize(img, (500, 300)) print(img_resized1.shape) cv2.imshow("sample001", img_resized1) cv2.waitKey(0) cv2.destroyAllWindows() # 画像のサイズ変更(倍率指定) 1倍⇒1.0 1/10倍⇒0.1 1.25倍⇒1.25 # サイズの形状は、縦×横だが、サイズ変更時は、横×縦で指定する形となる。 img_resized2 = cv2.resize(img, None, fx=0.1, fy=0.1) # 縮小 # img_resized2 = cv2.resize(img, None, fx=1.25, fy=1.25) # 拡大 print(img_resized2.shape) cv2.imshow("sample001", img_resized2) cv2.waitKey(0) cv2.destroyAllWindows()
画像の回転
画像を回転する方法。
# 画像の回転90度 img_rotated = cv2.rotate(img, cv2.ROTATE_90_CLOCKWISE) print(img_rotated.shape) cv2.imshow("sample004", img_rotated) cv2.waitKey(0) cv2.destroyAllWindows() # 画像の45度回転 # 画像の中心を求める height, width = img.shape[:2] center = (int(width/2), int(height/2)) # 画像を45度回転させる rot = cv2.getRotationMatrix2D(center, 45, 1) img_rotated = cv2.warpAffine(img, rot, (width,height)) print(img_rotated.shape) cv2.imshow("sample005", img_rotated) cv2.waitKey(0) cv2.destroyAllWindows() # 画像の上下左右反転 # 上下反転 img_reverse =cv2.flip(img, 0) print(img_reverse.shape) cv2.imshow("sample006", img_reverse) cv2.waitKey(0) cv2.destroyAllWindows() # 左右反転 img_reverse =cv2.flip(img, 1) print(img_reverse.shape) cv2.imshow("sample007", img_reverse) cv2.waitKey(0) cv2.destroyAllWindows() # 上下左右反転 img_reverse =cv2.flip(img, -1) print(img_reverse.shape) cv2.imshow("sample008", img_reverse) cv2.waitKey(0) cv2.destroyAllWindows()
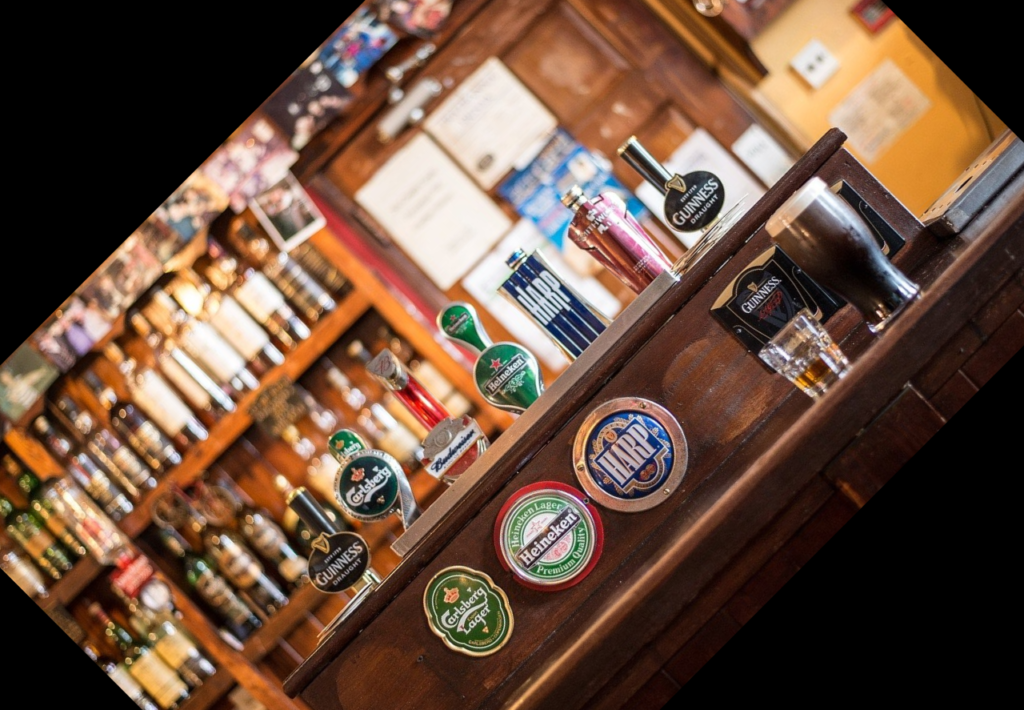
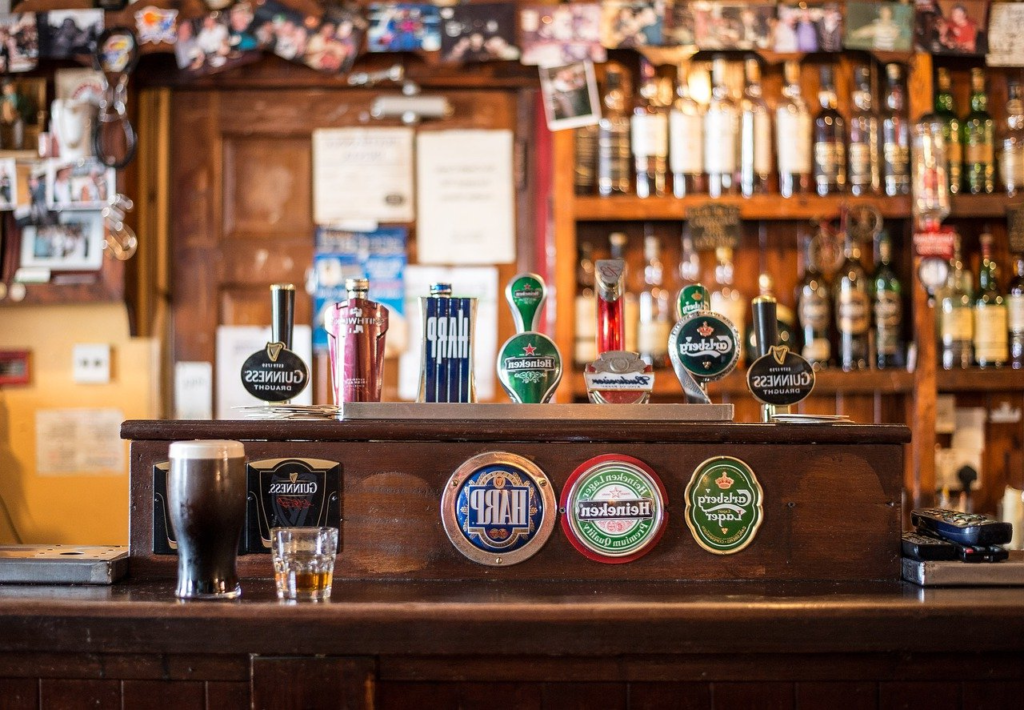
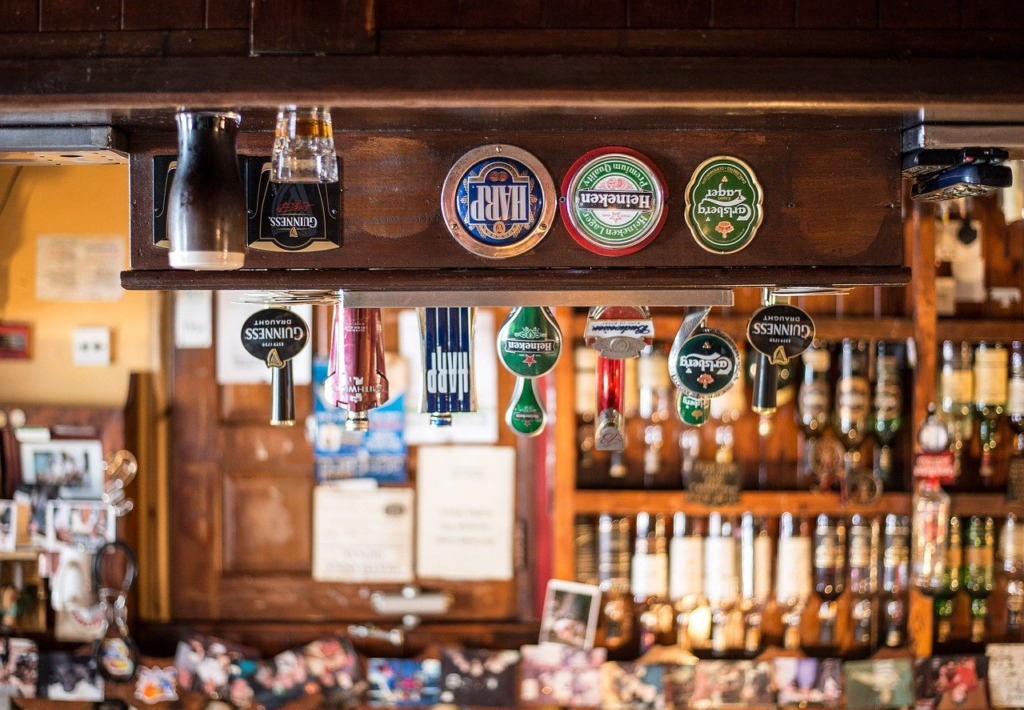